In the first article, we introduced Python strings and covered some of the basics. In this article, we will continue our look at strings. Escape Sequences In the last article, we introduced the following example: >>> 'string\'s', … [Continue reading]
Python Strings: Part One
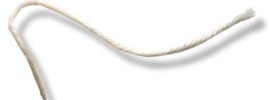
Introduction to Python Strings A string in Python is an ordered collection of characters used to store and represent text-based information. From a functional perspective, strings can be used to represent just about anything that can be encoded as … [Continue reading]
Python Exceptions: Part Six
As a special case for debugging purposes, Python includes the assert statement; it can be thought of as a conditional raise statement. A statement of the form: assert , <test> <data> works like the following code: if … [Continue reading]
Python Exceptions: Part Five
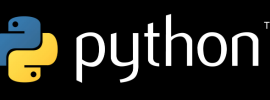
The Raise Statement One of the statements not yet covered in this series on exceptions is the raise statement. To trigger excepts explicitly in Python, you can code raise statements. Their general form is simple - a raise statement consists of the … [Continue reading]
Python Exceptions: Part Four
Python Exceptions: try/except/finally In all versions of Python prior to Release 2.5, there were two types of try statements. You could either use a finally to ensure that cleanup code was always run, or write except blocks to catch and recover … [Continue reading]
Python Exceptions: Part Three
In the previous articles, we covered the try and except clauses. In this article, we will start out by looking at the else clause. Python Exceptions: The Else Clause Although the purpose of the else clause may not be readily apparent, it is … [Continue reading]
Python Exceptions: Part Two
In the previous article, we covered some of the basics of exception handling in Python. In this article, we will go into a bit more detail on exception processing, and explore the details behind the try, raise, assert, and with statements. Python … [Continue reading]
Python Exceptions: Part One
Exceptions are events that can modify the flow of control through a program; they are triggered automatically on errors, and can be triggered and intercepted by your Python code. Python has the following statements to deal with … [Continue reading]
matplotlib: Part Two
In the previous article, I introduced matplotlib and some basic pylab statements that can be used to produce graphs. In this article, we'll use matplotlib and some basic computation to understand experimental data. numpy Arrays One of the examples … [Continue reading]
matplotlib: Part One
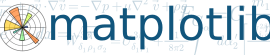
matplotlib is a plotting library for the Python programming language and its numerical mathematics extension NumPy. It provides an object-oritented API for embedding plots into applications using general-purpose GUI toolkits. Since it is designed … [Continue reading]
Recent Comments